pwd
— print the current directorycd folder
— change the current directory to folder
dir
— list contents of the current directorygit config
command. Git will use these details to assign version authorship.git config --global user.name "Tibor Stanko"
git config --global user.email "[email protected]"
main
(we will talk more about branches later)git init
command is used to create a Git repository in the current directory:git status
:Saving a version involves two steps.
git add
to mark changes that should be added to the new version:git commit
to create a record of the new version:-m "commit message"
argument.-m
argument. git commit
will then open a text editor where you can write the description.vim
, which runs directly in the terminal. If you are not familiar with using vim
, you can change the editor:Don’t slack off when writing commit messages!
Some version control systems work by creating a new version from all the current files in the repository. This method of saving backups can be inefficient. For example, if we have implemented two independent features in the repository and we want to capture them in two separate versions. Therefore, Git introduces the concept of the staging area, which allows us to control which changes will be included in the next version.
git status
:git log
command to verify that a commit was created:commit bf5c9b4a320012b422546fcb86f5b957104bea55 (HEAD -> main)
Author: Tibor Stanko <[email protected]>
Date: Tue Sep 13 17:00:00 2022 +0200
Add hello.py
git reset [file]
to unstage a file while retaining changes in the working directorygit reset [file]
to unstage a file while retaining changes in the working directoryzoo
on your computer.zoo
directory into a Git repository.test.txt
with any content.test.txt
to the next version and save it.git log
.Tip: don’t forget to use git status
while working to see the current status of the repository.
Branches allow us to deviate from the main line and continue working without interfering with it
Branching is a strong feature of Git — switching between branches is fast, which allows for frequent creation of new branches
So far, we have been working on the main
branch, which was automatically created by git init
We can see a list of branches using the git branch
command:
The default Git branch used to be master
. Due to its negative connotations, this name is gradually being phased out. Therefore, when configuring Git, we changed init.defaultBranch
to main
.
rewrite/improve second bullet
french
, we call:slovak
branch to the main main
branch, we first switch to the main branch:git merge
command to merge branches:>> git merge french
Auto-merging hello.py
CONFLICT (content): Merge conflict in hello.py
Automatic merge failed; fix conflicts and then commit the result.
git branch --delete
, or git branch -d
for short.After deletion, the branch is removed from history and cannot be restored.
git log
historyadd mermaid diagram for this git log output
animals
in your local repository.zoo.txt
in the repository with the following content:zoo.txt
.animals
branch into the main
branch and delete the animals
branch.tiger
branch, change the lev
line to tiger
and save a new version.main
branch, fix the zirafa
line to žirafa
, and save a new version.tiger
branch into the main
branch.So far, we have been working with a local Git repository that is stored on our computer
A remote repository is stored on the Internet — more precisely, on a web server
e.g. github.com, corporate server, university server, …
git remote add <name> <url>
command to set up a remote repository:name
is used by Git as the name of the remote repository at url
. The name can be anything; the name origin
is commonly used.git push <remote> <branch>
command “pushes” local changes from the branch
branch to the remote repository remote
:git push
for the first time, you need to add the -u
argument:-u
or --set-upstream
sets the default remote branch (origin/main
) for the current local branch (main
)
if the remote branch origin/main
does not exist, git push
will create it automatically
git push
git push
is rungit branch -a
lists all branches, both local and remote-a
is short for --all
*
indicates the current branch:To https://github.com/bbrrck/zoo.git
! [rejected] main -> main (fetch first)
error: failed to push some refs to 'https://github.com/bbrrck/zoo.git'
hint: Updates were rejected because the remote contains work that you do
hint: not have locally. This is usually caused by another repository pushing
hint: to the same ref. You may want to first integrate the remote changes
hint: (e.g., 'git pull ...') before pushing again.
hint: See the 'Note about fast-forwards' in 'git push --help' for details.
git fetch
command to fetch the list of changes from the remote branch:git merge
:git pull
command, which is a combination of git fetch
and git merge
:git clone
:remote_url
in the local_folder
directoryzoo
.main
branch to the remote.zoo.txt
file: 🐼🐘🐯🦒.krokodíl 🐊
to the zoo.txt
file and save a new version.gorila 🦍
to zoo.txt
and save a new version..gitignore
git <command> --help
git diff
git cat-file -p
git restore
git add --interactive
git log
git stash
git blame
git revert
The git revert
command creates a new version, and does not modify the history of the repository.
git reset
The git reset
command modifies the history of the repository and can cause file loss.
README.md
file that Github automatically rendersREADME
files: matiassingers/awesome-readmeEven these slides were created using Markdown! (with the help of the Quarto system)
# Markdown is Awesome Markdown is very simple and versatile. This is a Markdown paragraph. This is still the same paragraph. ## Formatting options Bulleted list: - *italic* - **bold** - ***bold and italic*** - ~~strikethrough~~ - [link](https://www.markdownguide.org/) - `code` Numbered list: 1. first item 2. second item 3. last item
Markdown is very simple and versatile.
This is a Markdown paragraph. This is still the same paragraph.
Bulleted list:
code
Numbered list:
### Code blocks ```python def main(): print("hello!") if __name__ == "__main__": main() ``` ### Images 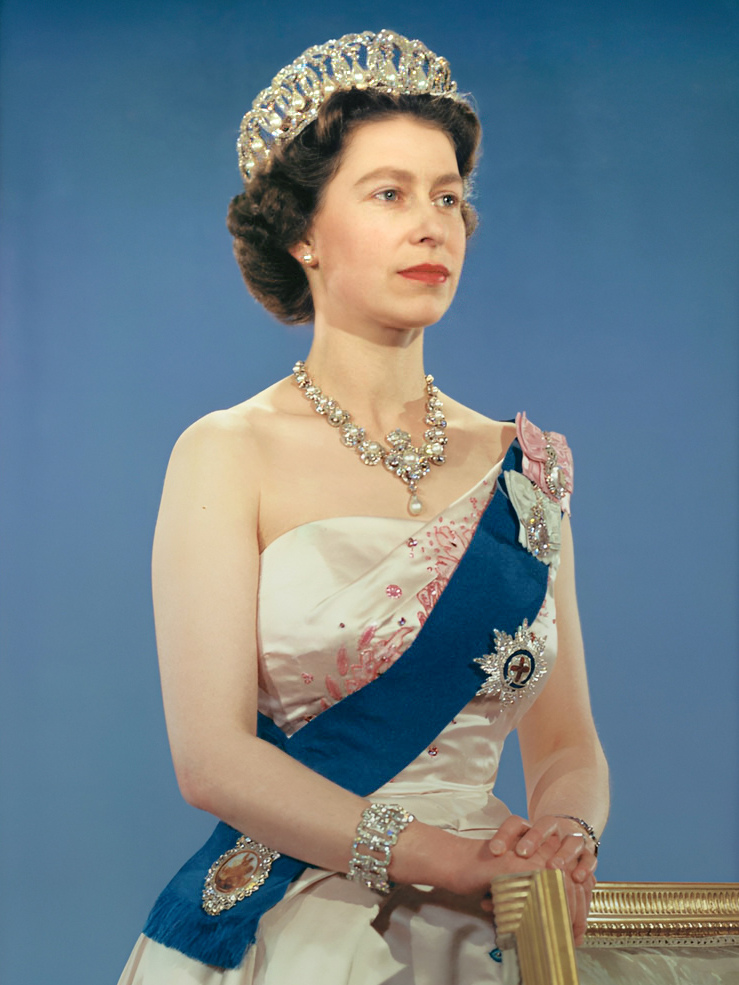 ### Blockquotes > It’s worth remembering that it is often the small steps, not the giant leaps, that bring about the most lasting change.
en | sk |
---|---|
branch | vetva |
clone | naklonovanie repozitára |
commit | záznam |
commit message | popis záznamu |
conflict | konflikt medzi verziami |
conflict resolution | riešenie konfliktov |
diff | rozdiel medzi verziami |
merge | zlúčenie vetiev |
en | sk |
---|---|
pull | stiahnutie vzdialených zmien |
push | odoslanie lokálnych zmien |
repository | repozitár, úložisko |
remote | vzdialený repozitár |
snapshot | snímka |
staging area | prípravná oblasť (tiež index) |
status | stav repozitára |
version | verzia |